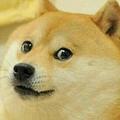
dtonhofer
Functional Programming in Java, Second Edition: Chapter 12
On page 200, the code can use boxed()
instead of the (somewhat mysterious) maptoObject(e -> e)
One should also reveal to the reader that we are computing perfect numbers
package chapter12;
import org.junit.jupiter.api.Test;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.LongStream;
public class Chapter12 {
// Confusing code on page 200
// but with mapToObj(e -> e) replaced with boxed()
private static List<Long> confusingCode(long number) {
return LongStream.rangeClosed(1, number)
.filter(i -> { //Bad Code, don't do this
long factor = 0;
for (int j = 1; j < i; j++) {
if (i % j == 0) {
factor += j;
}
}
return factor == i;
})
.boxed()
.toList();
}
// Confusing code on page 201
// but with mapToObj(e -> e) replaced with boxed()
private static List<Long> refactoredConfusingCode(long number) {
return LongStream.range(1, number)
.filter(i -> LongStream.range(1, i) //Not good
.filter(j -> i % j == 0)
.sum() == i)
.boxed()
.toList();
}
// Nonconfusing code on page 201, in two methods
private static Long sumOfDivisors(long number) {
return LongStream.range(1, number)
.filter(i -> number % i == 0)
.sum();
}
// Create list of those x <= limit such that the sum of the divisors of x equals x
// https://en.wikipedia.org/wiki/Perfect_number
// https://oeis.org/A000396
private static List<Long> nonConfusingCode(long limit) {
return LongStream.rangeClosed(1, limit)
.filter(i -> sumOfDivisors(i) == i)
.boxed()
.toList();
}
// A record to pass data along the stream
private record InAndOut(Long in, List<Long> out) {
public String toString() {
return "f(" + in + ")=[" + out.stream().map(it -> Long.toString(it)).collect(Collectors.joining(",")) + "]";
}
}
private static String exerciseCode(Function<Long, List<Long>> f, List<Long> input) {
return input.stream()
.map(it -> new InAndOut(it, f.apply(it)))
.map(InAndOut::toString)
.collect(Collectors.joining(", "));
}
@Test
public void exerciseAllCode() {
var input = List.of(0L, 1L, 2L, 10L, 13L, 100L, 1000L, 10000L);
{
String str = exerciseCode(Chapter12::confusingCode, input);
System.out.println(str);
}
{
String str = exerciseCode(Chapter12::refactoredConfusingCode, input);
System.out.println(str);
}
{
String str = exerciseCode(Chapter12::nonConfusingCode, input);
System.out.println(str);
}
}
}
Popular Pragmatic Bookshelf topics
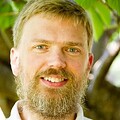
Some minor things in the paper edition that says “3 2020” on the title page verso, not mentioned in the book’s errata online:
p. 186 But...
New
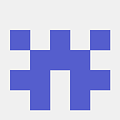
Title: Web Development with Clojure, Third Edition, pg 116
Hi - I just started chapter 5 and I am stuck on page 116 while trying to star...
New
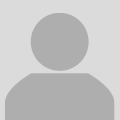
Hello! On page xix of the preface, it says there is a community forum "… for help if your’re stuck on one of the exercises in this book… ...
New
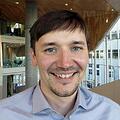
On the page xv there is an instruction to run bin/setup from the main folder. I downloaded the source code today (12/03/21) and can’t see...
New
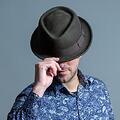
In case this helps anyone, I’ve had issues setting up the rails source code. Here were the solutions:
In Gemfile, change
gem 'rails'
t...
New
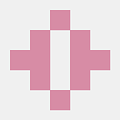
It seems the second code snippet is missing the code to set the current_user:
current_user: Accounts.get_user_by_session_token(session["...
New
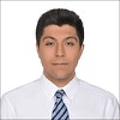
AWDWR 7, page 152, page 153:
Hello everyone,
I’m a little bit lost on the hotwire part. I didn’t fully understand it.
On page 152 @rub...
New
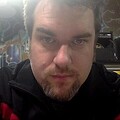
Docker-Machine became part of the Docker Toolbox, which was deprecated in 2020, long after Docker Desktop supported Docker Engine nativel...
New
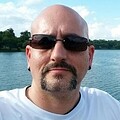
root_layout: {PentoWeb.LayoutView, :root},
This results in the following following error:
no “root” html template defined for PentoWeb...
New
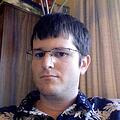
@mfazio23
Android Studio will not accept anything I do when trying to use the Transformations class, as described on pp. 140-141. Googl...
New
Other popular topics
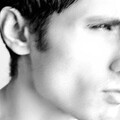
There’s a whole world of custom keycaps out there that I didn’t know existed!
Check out all of our Keycaps threads here:
https://forum....
New
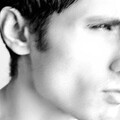
I’ve been hearing quite a lot of comments relating to the sound of a keyboard, with one of the most desirable of these called ‘thock’, he...
New
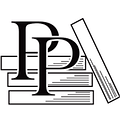
Rust is an exciting new programming language combining the power of C with memory safety, fearless concurrency, and productivity boosters...
New
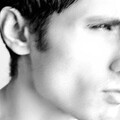
Just done a fresh install of macOS Big Sur and on installing Erlang I am getting:
asdf install erlang 23.1.2
Configure failed.
checking ...
New
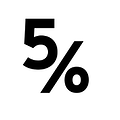
Here’s the story how one of the world’s first production deployments of LiveView came to be - and how trying to improve it almost caused ...
New
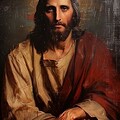
The File System Access API with Origin Private File System.
WebKit supports new API that makes it possible for web apps to create, open,...
New
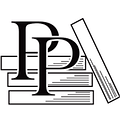
Author Spotlight
Jamis Buck
@jamis
This month, we have the pleasure of spotlighting author Jamis Buck, who has written Mazes for Prog...
New
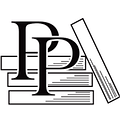
Author Spotlight:
Tammy Coron
@Paradox927
Gaming, and writing games in particular, is about passion, vision, experience, and immersio...
New
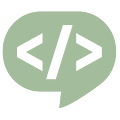
Will Swifties’ war on AI fakes spark a deepfake porn reckoning?
New
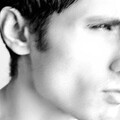
If you’re getting errors like this:
psql: error: connection to server on socket “/tmp/.s.PGSQL.5432” failed: No such file or directory ...
New
Latest in Functional Programming in Java, Second Edition
Latest in PragProg Customers
Latest (all)
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /js
- /rails
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /haskell
- /emacs
- /svelte
- /onivim
- /typescript
- /crystal
- /c-plus-plus
- /tailwind
- /kotlin
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /vscode
- /opensuse
- /ash
- /centos
- /php
- /deepseek
- /scala
- /zig
- /html
- /debian
- /nixos
- /lisp
- /agda
- /react-native
- /textmate
- /sublime-text
- /kubuntu
- /arch-linux
- /ubuntu
- /revery
- /manjaro
- /django
- /spring
- /diversity
- /nodejs
- /lua
- /julia
- /slackware
- /c
- /neovim