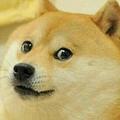
dtonhofer
Functional Programming in Java, Second Edition: Functional Programming in Java, Second Edition: JUnit code improvements for Chapter 11, pages 195 ff “Refactoring Nested Loops"
Nothing surprising here, just adapting the form to the other examples.
Ah yes, compute()
now returns an immutable list in all cases.
package chapter11;
import org.junit.jupiter.api.Test;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.stream.IntStream;
import java.util.stream.Stream;
import static org.junit.jupiter.api.Assertions.assertAll;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class NestedLoopTest {
record Triple(int a, int b, int c) {
public String toString() {
return String.format("%d %d %d", a, b, c);
}
}
private static Triple triple(int a, int b, int c) {
return new Triple(a, b, c);
}
interface PythagoreanTriples {
List<Triple> compute(int numberOfValues);
}
// (m² - n², 2 * m * n, m² + n²) is a valid triple because, resolving
// (m⁴ + n⁴ - 2 * m² * n²) + (4 * m² * n²) = m⁴ + n⁴ + 2 * m² * n²
// assuming m > n
private static Triple getTripleEuclidsWay(final int m, final int n) {
if (m <= n) {
throw new IllegalArgumentException("m <= n");
}
final int a = m * m - n * n;
final int b = 2 * m * n;
final int c = m * m + n * n;
return triple(a, b, c);
}
static class PythagoreanTriplesBefore implements PythagoreanTriples {
public List<Triple> compute(int tripleCount) {
if (tripleCount == 0) {
return List.of(); // unmodifiable
}
List<Triple> triples = new ArrayList<>();
for (int m = 2; ; m++) {
for (int n = 1; n < m; n++) {
triples.add(getTripleEuclidsWay(m, n));
if (triples.size() == tripleCount)
break;
}
if (triples.size() == tripleCount)
break;
}
return Collections.unmodifiableList(triples);
}
}
static class PythagoreanTriplesAfter implements PythagoreanTriples {
public List<Triple> compute(int tripleCount) {
return Stream.iterate(2, e -> e + 1)
.flatMap(m -> IntStream.range(1, m).mapToObj(n -> getTripleEuclidsWay(m, n)))
.limit(tripleCount)
.toList();
}
}
private static void commonPythagoreanTriplesTests(final PythagoreanTriples pytris) {
assertAll(
() -> assertEquals(List.of(), pytris.compute(0)),
() -> assertEquals(List.of(triple(3, 4, 5)),
pytris.compute(1)),
() -> assertEquals(
List.of(triple(3, 4, 5), triple(8, 6, 10), triple(5, 12, 13)),
pytris.compute(3)),
() -> assertEquals(
List.of(triple(3, 4, 5), triple(8, 6, 10),
triple(5, 12, 13), triple(15, 8, 17),
triple(12, 16, 20)),
pytris.compute(5))
);
}
@Test
void pythagoreanTriplesBefore() {
commonPythagoreanTriplesTests(new PythagoreanTriplesBefore());
}
@Test
void pythagoreanTriplesAfter() {
commonPythagoreanTriplesTests(new PythagoreanTriplesAfter());
}
}
Popular Pragmatic Bookshelf topics
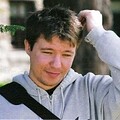
In Chapter 3, the source for index introduces Config on page 31, followed by more code including tests; Config isn’t introduced until pag...
New
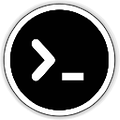
Title: Design and Build Great Web APIs - typo “https://company-atk.herokuapp.com/2258ie4t68jv” (page 19, third bullet in URL list)
Typo:...
New
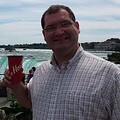
When I try the command to create a pair of migration files I get an error.
user=> (create-migration "guestbook")
Execution error (Ill...
New
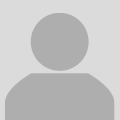
Hi Travis! Thank you for the cool book! :slight_smile:
I made a list of issues and thought I could post them chapter by chapter. I’m rev...
New
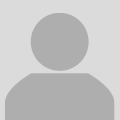
This isn’t directly about the book contents so maybe not the right forum…but in some of the code apps (e.g. turbo/06) it sends a TURBO_ST...
New
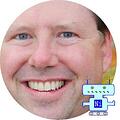
I am working on the “Your Turn” for chapter one and building out the restart button talked about on page 27. It recommends looking into ...
New
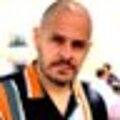
On page 78 the following code appears:
<%= link_to ‘Destroy’, product,
class: ‘hover:underline’,
method: :delete,
data: { confirm...
New
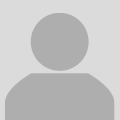
When running the program in chapter 8, “Implementing Combat”, the printout Health before attack was never printed so I assumed something ...
New
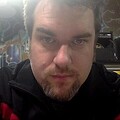
Docker-Machine became part of the Docker Toolbox, which was deprecated in 2020, long after Docker Desktop supported Docker Engine nativel...
New
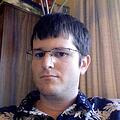
I’ve got to the end of Ch. 11, and the app runs, with all tabs displaying what they should – at first. After switching around between St...
New
Other popular topics
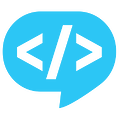
Reading something? Working on something? Planning something? Changing jobs even!?
If you’re up for sharing, please let us know what you’...
New
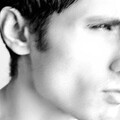
I have seen the keycaps I want - they are due for a group-buy this week but won’t be delivered until October next year!!! :rofl:
The Ser...
New
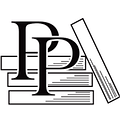
“Finding the Boundaries” Hero’s Journey with Noel Rappin @noelrappin
Even when you’re ultimately right about what the future ho...
New
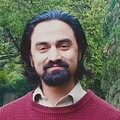
The V Programming Language
Simple language for building maintainable programs
V is already mentioned couple of times in the forum, but I...
New
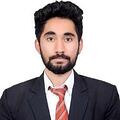
API 4
Path:
/user/following/
Method:
GET
Description:
Returns the list of all names of people whom the user follows
Response
[
{ ...
New
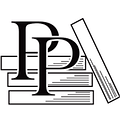
Author Spotlight
Erin Dees
@undees
Welcome to our new author spotlight! We had the pleasure of chatting with Erin Dees, co-author of ...
New
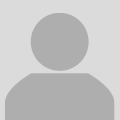
Inside our android webview app, we are trying to paste the copied content from another app eg (notes) using navigator.clipboard.readtext ...
New
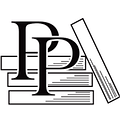
Author Spotlight:
Karl Stolley
@karlstolley
Logic! Rhetoric! Prag! Wow, what a combination. In this spotlight, we sit down with Karl ...
New
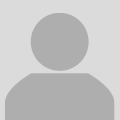
There appears to have been an update that has changed the terminology for what has previously been known as the Taskbar Overflow - this h...
New
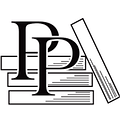
Author Spotlight:
Tammy Coron
@Paradox927
Gaming, and writing games in particular, is about passion, vision, experience, and immersio...
New
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /emacs
- /haskell
- /java
- /onivim
- /svelte
- /typescript
- /crystal
- /kotlin
- /c-plus-plus
- /tailwind
- /gleam
- /ocaml
- /react
- /elm
- /flutter
- /vscode
- /ash
- /opensuse
- /centos
- /html
- /php
- /deepseek
- /zig
- /scala
- /lisp
- /textmate
- /sublime-text
- /debian
- /nixos
- /react-native
- /agda
- /kubuntu
- /arch-linux
- /revery
- /django
- /ubuntu
- /spring
- /manjaro
- /diversity
- /nodejs
- /lua
- /c
- /slackware
- /julia
- /markdown