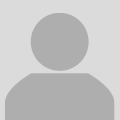
Aathithyan
I don't know how to implement outgoing call feature in android studio using Kotlin
I need to know how to implement outgoing call in my custom application instead of using default phone call app i need my own app call.
Most Liked
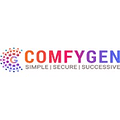
comfygenpvt
Implementing outgoing calls in a custom Android application involves several steps. Below is a basic outline to guide you through the process. Note that this is a simplified overview, and you may need to adjust the code based on your specific requirements.
Add Permissions: Ensure that you have the necessary permissions in your AndroidManifest.xml file:
<uses-permission android:name=android.permission.CALL_PHONE" />
Create UI for Dialing: Design a user interface (UI) for dialing a phone number. You can use EditText
for the phone number input and a Button
to trigger the call.
<EditText
android:id="@+id/editTextPhoneNumber"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="phone" />
<Button
android:id="@+id/buttonCall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Call" />
Handle Call Button Click: In your activity or fragment, handle the button click event and initiate the call:
Button buttonCall = findViewById(R.id.buttonCall);
buttonCall.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String phoneNumber = ((EditText) findViewById(R.id.editTextPhoneNumber)).getText().toString();
makePhoneCall(phoneNumber);
}
});
Implement makePhoneCall
Method: Create a method to initiate the phone call using an Intent
. This will launch the default phone app with the specified phone number:
private void makePhoneCall(String phoneNumber) {
Intent dialIntent = new Intent(Intent.ACTION_CALL, Uri.parse("tel:" + phoneNumber));
startActivity(dialIntent);
}
Note: Starting from Android 23 (Marshmallow), you need to request the CALL_PHONE
permission at runtime. You can check for permission before making the call and request it if necessary.
if (ContextCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) == PackageManager.PERMISSION_GRANTED) {
makePhoneCall(phoneNumber);
} else {
// Request permission
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.CALL_PHONE}, CALL_PHONE_PERMISSION_REQUEST_CODE);
}
Handle the permission result in the onRequestPermissionsResult
method.
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
if (requestCode == CALL_PHONE_PERMISSION_REQUEST_CODE) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
makePhoneCall(phoneNumber);
} else {
Toast.makeText(this, "Call permission denied", Toast.LENGTH_SHORT).show();
}
}
}
Remember to replace CALL_PHONE_PERMISSION_REQUEST_CODE
with a constant value defined in your code.
Please note that the CALL_PHONE
permission allows your app to make calls directly without user interaction. Ensure that you comply with privacy and security guidelines when implementing such features in your application.
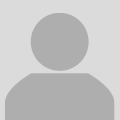
Veeran
To implement outgoing calls in your custom application, you can use Android’s TelephonyManager and Intent classes. First of all, request the necessary permissions in your AndroidManifest.xml. Then, use TelephonyManager to check if the device is capable of making calls. If yes, use Intent.ACTION_CALL to initiate an outgoing call with the desired phone number. Also, ensure you handle the appropriate permissions and runtime checks to avoid issues.
Regards
Popular Android topics
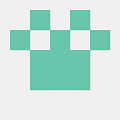
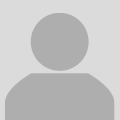
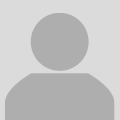
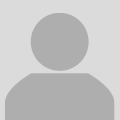
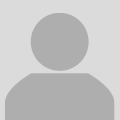
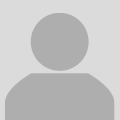
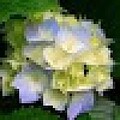
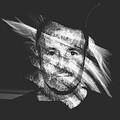
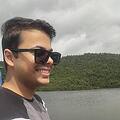
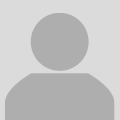
Other popular topics
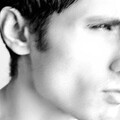
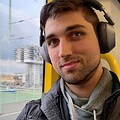
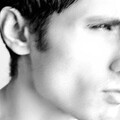
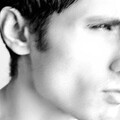
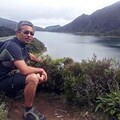
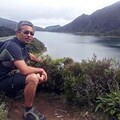
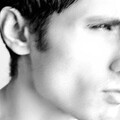
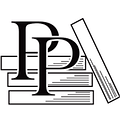
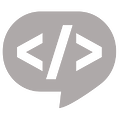
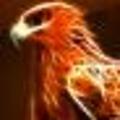
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /ruby
- /wasm
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /emacs
- /haskell
- /java
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /ocaml
- /react
- /elm
- /flutter
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /sublime-text
- /lisp
- /textmate
- /debian
- /nixos
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /django
- /ubuntu
- /manjaro
- /spring
- /nodejs
- /diversity
- /lua
- /julia
- /c
- /slackware
- /markdown