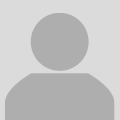
harwind
Python concerns with the For Loop and While Loop
Hi, I’m now investigating the complexities of Python loops, specifically the contrast between for and while loops. However, I’ve had some difficulty understanding their distinctions and implementing them optimally. Here are some bits of code that demonstrate my areas of confusion.
Snippet 1:
# Using a for loop to iterate over a string
word = "Python"
for letter in word:
print(letter)
Snippet 2:
# Using a while loop to iterate over a string
word = "Python"
length = len(word)
i = 0
while i < length:
print(word[i])
i += 1
Here are the specific topics I need explanation on:
- While Snippet 1 (using a for loop) displays each letter of the word “Python” consecutively, Snippet 2 (using a while loop) produces the same output but does not include the last character ‘n’. What is causing this disparity, and how can I change the while loop to incorporate the last character?
- While considering the readability and simplicity of both loops, I’m not sure which strategy is best for iterating over strings in Python. Are there any rules or best practices for deciding between for and while loops when working with strings?
- In terms of efficiency, as demonstrated in this documentation, I’m interested about the overhead of using len() in the while loop to ascertain string length. What effect does this have on the loop’s efficiency when compared to the for loop’s implicit iteration?
- Finally, I’d like to understand the circumstances in which each loop type excels in Python programming. Can you give instances or insights into circumstances when for loops or while loops are very useful for certain tasks?
Your experience and instruction would be extremely helpful in navigating these complexities and improving my skill with Python loop constructions. Thank you for your help.
Popular General Dev topics
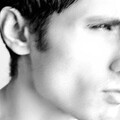
This article got me thinking about encrypted chat:
Europol said that French police had discovered some of EncroChat’s servers were lo...
New
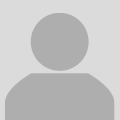
What are the main stages of software development?
New
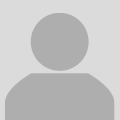
How can I apply a modified date and time to a variable? This is what I get when I execute the following query in SQL Server Mgmt Studio: ...
New
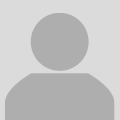
Hello devtalk !
Heroku are closing their free tier (dynos) later this month and I was wondering if you guys could recommend any alternat...
New
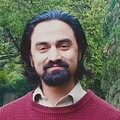
I have always used antique keyboards like Cherry MX 1800 or Cherry MX 8100 and almost always have modified the switches in some way, like...
New
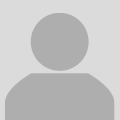
Consider the following bits of code:
void foo(const int i) // First foo
{
std::cout << "First " << i << endl;
}
vo...
New
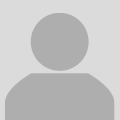
I have an array of objects in JavaScript, and I want to sort them based on a specific property of the objects. For example, I have an arr...
New
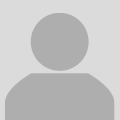
Given an array of integers, find the length of the longest increasing subsequence. A subsequence is a sequence that can be derived from a...
New
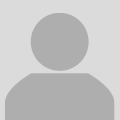
Hello,
I am new to this forum. Not really sure if this topic is relevant for this chat at all. I apologize if its not.
I am trying to c...
New
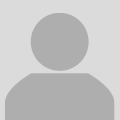
Hello everyone! I am not a developer, just wanna know if it’s possible for someone with no skills to learn how to reverse hack my hackers.
New
Other popular topics
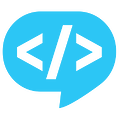
Hello Devtalk World!
Please let us know a little about who you are and where you’re from :nerd_face:
New
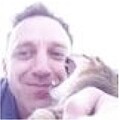
Any thoughts on Svelte?
Svelte is a radical new approach to building user interfaces. Whereas traditional frameworks like React and Vue...
New
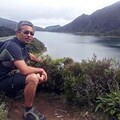
Please tell us what is your preferred monitor setup for programming(not gaming) and why you have chosen it.
Does your monitor have eye p...
New
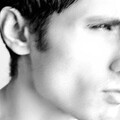
Thanks to @foxtrottwist’s and @Tomas’s posts in this thread: Poll: Which code editor do you use? I bought Onivim! :nerd_face:
https://on...
New
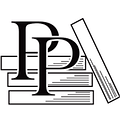
Learn different ways of writing concurrent code in Elixir and increase your application's performance, without sacrificing scalability or...
New
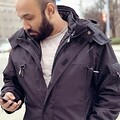
Crystal recently reached version 1. I had been following it for awhile but never got to really learn it. Most languages I picked up out o...
New
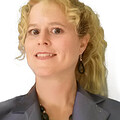
Hello everyone! This thread is to tell you about what authors from The Pragmatic Bookshelf are writing on Medium.
New
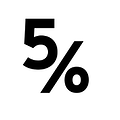
Here’s the story how one of the world’s first production deployments of LiveView came to be - and how trying to improve it almost caused ...
New
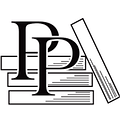
Build efficient applications that exploit the unique benefits of a pure functional language, learning from an engineer who uses Haskell t...
New
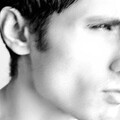
Was just curious to see if any were around, found this one:
I got 51/100:
Not sure if it was meant to buy I am sure at times the b...
New
Categories:
Sub Categories:
- All
- In The News (10018)
- Dev Chat (200)
- Questions
- Resources (118)
- Blogs/Talks (26)
- Jobs (3)
- Events (15)
- Code Editors (58)
- Hardware (57)
- Reviews (4)
- Sales (15)
- Design & UX (4)
- Marketing & SEO (1)
- Industry & Culture (14)
- Ethics & Privacy (19)
- Business (4)
- Learning Methods (4)
- Content Creators (7)
- DevOps & Hosting (9)
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /haskell
- /emacs
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /tailwind
- /kotlin
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /ash
- /vscode
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /debian
- /nixos
- /textmate
- /sublime-text
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /ubuntu
- /revery
- /spring
- /manjaro
- /django
- /diversity
- /nodejs
- /lua
- /slackware
- /julia
- /c
- /markdown