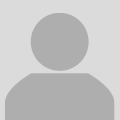
harwind
JavaScript Challenge: Validating Email Addresses
I’m working on a web application where users can sign up with their email addresses. To ensure data integrity, I want to implement client-side validation to check if the email address provided by the user is in a valid format.
Here’s a simplified version of my JavaScript code:
function validateEmail(email) {
// Regular expression to validate email format
var regex = /* Regular expression goes here */;
if (regex.test(email)) {
return true;
} else {
return false;
}
}
var userEmail = "user@example.com";
var isValid = validateEmail(userEmail);
if (isValid) {
console.log("Email is valid.");
} else {
console.log("Email is not valid.");
}
In this code, I have a validateEmail
function that should return true
if the email
parameter matches a valid email format and false
otherwise. However, I’m not sure what regular expression to use to validate email addresses correctly.
Could you provide a JavaScript code example that includes a regular expression for validating email addresses? Additionally, it would be helpful if you could explain how the regular expression works and any considerations for email validation in JavaScript. Thank you for your assistance!
Most Liked
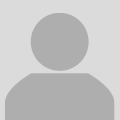
Bleep9279
Not sure if this is applicable to your scenario, but if you’re validating user input, you can use type="email"
and make this the browser’s problem.
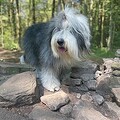
mindriot
According to the docs, browsers use an algorithm equivalent to this regex for email input types:
/^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/;
So that could be a reasonably good baseline generally especially if you want to use those inputs at some point. However this does only check that the email address is of a valid format rather than necessarily meaning it is actually a valid email address, for that you likely need to go down the route of sending a validation link to the email address. It is also worth keeping in mind that if you are only doing this check in the client side then it will still be possible for someone to send messed up data directly to the server.
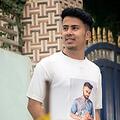
gulshan212
Can you try below code, I am sure you can get what you are looking for.
function validateEmail(email) {
// Regular expression to validate email format
var regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
if (regex.test(email)) {
return true;
} else {
return false;
}
}
var userEmail = "user@example.com";
var isValid = validateEmail(userEmail);
if (isValid) {
console.log("Email is valid.");
} else {
console.log("Email is not valid.");
}
Thanks
Popular General Dev topics
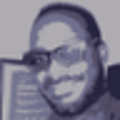
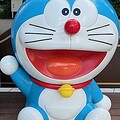
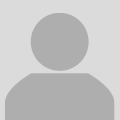
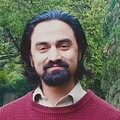
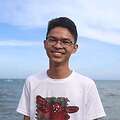
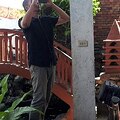
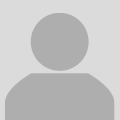
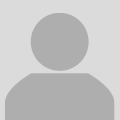
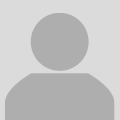
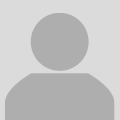
Other popular topics
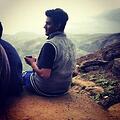
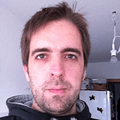
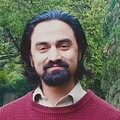
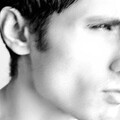
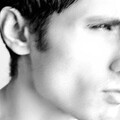
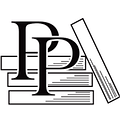
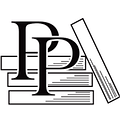
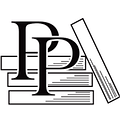
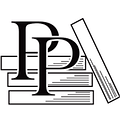
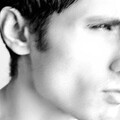
Categories:
Sub Categories:
- All
- In The News (10018)
- Dev Chat (200)
- Questions
- Resources (118)
- Blogs/Talks (26)
- Jobs (3)
- Events (15)
- Code Editors (58)
- Hardware (57)
- Reviews (4)
- Sales (15)
- Design & UX (4)
- Marketing & SEO (1)
- Industry & Culture (14)
- Ethics & Privacy (19)
- Business (4)
- Learning Methods (4)
- Content Creators (7)
- DevOps & Hosting (9)
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /haskell
- /emacs
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /ash
- /vscode
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /scala
- /zig
- /debian
- /nixos
- /textmate
- /sublime-text
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /ubuntu
- /revery
- /manjaro
- /spring
- /django
- /diversity
- /lua
- /nodejs
- /c
- /julia
- /slackware
- /neovim