Navigating the Maze of Exception Handling in Java Programs
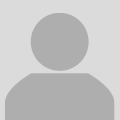
Hi,
Take a riveting look at exception handling in Java programming, including the complicated dance between try-catch blocks, checked and unchecked exceptions, and exception propagation techniques. This topic digs into the difficulties of managing unusual cases, identifying frequent dangers, and developing robust error-handling mechanisms to protect Java programs from unanticipated events.
Scenario Overview:
In Java programming, exception management is a key component of robust software development, allowing developers to elegantly handle unexpected circumstances while maintaining application stability. From controlled exceptions that must be explicitly handled to unchecked exceptions that propagate up the call stack, knowing exception handling is critical for developing dependable and robust Java systems.
here is the code snippet:
// Example demonstrating exception handling in Java with errors
public class Main {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[5]); // Error: ArrayIndexOutOfBoundsException
} catch (Exception e) {
System.out.println("An error occurred: " + e.getMessage()); // Error: getMessage() method is undefined
}
}
}
Key Points of Discussion:
Exception Types and Categories: Explore the taxonomy of exceptions in Java, distinguishing between checked exceptions (those that must be caught or declared) and unchecked exceptions (those that need not be explicitly handled). Examine the hierarchy of exception classes and their relationships, shedding light on common exceptions like NullPointerException and ArrayIndexOutOfBoundsException.
Handling Exceptions with try-catch Blocks: Delve into the syntax and semantics of try-catch blocks, where exceptional code segments are encapsulated within try blocks and handled within catch blocks. Investigate best practices for catching and handling exceptions effectively, ensuring graceful error recovery and preventing application crashes.
Exception Propagation and Throwing Exceptions: Learn about the methods that allow exceptions to propagate up the call stack until they are caught or reach the program’s top level. Investigate cases in which exceptions are thrown explicitly using the throw keyword, allowing developers to signal unusual events and initiate suitable error-handling algorithms, as illustrated below as an example.
Thank you
Hope someone will help
Popular General Dev topics
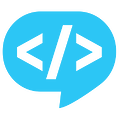
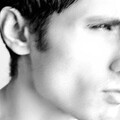
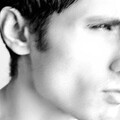
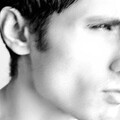
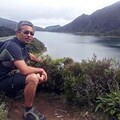
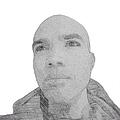
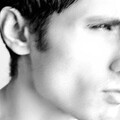
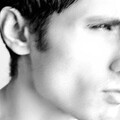
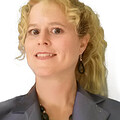
Other popular topics
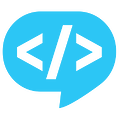
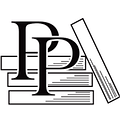
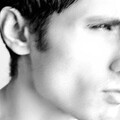
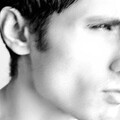
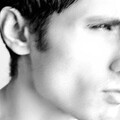
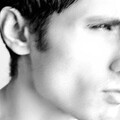
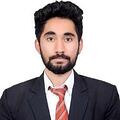
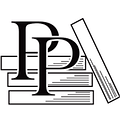
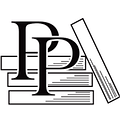
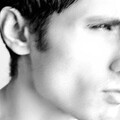
Latest in General Dev
Latest (all)
Categories:
My Saved Portals
-
None saved yet
Popular Portals
- /elixir
- /opensuse
- /rust
- /kotlin
- /ruby
- /erlang
- /python
- /clojure
- /react
- /quarkus
- /go
- /vapor
- /v
- /react-native
- /wasm
- /security
- /django
- /nodejs
- /centos
- /haskell
- /rails
- /fable
- /gleam
- /swift
- /js
- /deno
- /assemblyscript
- /tailwind
- /laravel
- /symfony
- /phoenix
- /crystal
- /typescript
- /debian
- /adonisjs
- /julia
- /arch-linux
- /svelte
- /spring
- /preact
- /flutter
- /c-plus-plus
- /actix
- /java
- /angular
- /ocaml
- /zig
- /kubuntu
- /scala
- /zotonic
- /vim
- /rocky
- /lisp
- /html
- /keyboards
- /nim
- /vuejs
- /emacs
- /elm
- /nerves