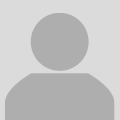
harwind
Dynamic Programming Challenge: Longest Increasing Subsequence
Given an array of integers, find the length of the longest increasing subsequence. A subsequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements.
For example:
#include <iostream>
#include <vector>
int longestIncreasingSubsequence(const std::vector<int>& nums) {
// Your dynamic programming solution goes here.
// Return the length of the longest increasing subsequence.
}
int main() {
std::vector<int> sequence = {10, 22, 9, 33, 21, 50, 41, 60, 80};
int result = longestIncreasingSubsequence(sequence);
std::cout << "Length of the longest increasing subsequence: " << result << std::endl;
return 0;
}
I’m specifically interested in implementing this using dynamic programming techniques. How can I approach this problem using dynamic programming, and what would be the C++ code for solving it? Any insights, code snippets, or explanations would be incredibly helpful in mastering dynamic programming for this particular challenge. Thank you for your assistance!
Popular General Dev topics
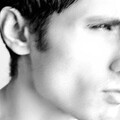
This article got me thinking about encrypted chat:
Europol said that French police had discovered some of EncroChat’s servers were lo...
New
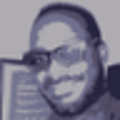
You can go directly to the last paragraph of this post to read about my concern.
I was trying Git submodules then found the above po...
New
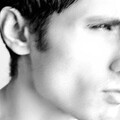
Stopwords are words that you normally filter for things like search queries, such as ‘as’, ‘because’ etc - there are a few online, but I ...
New
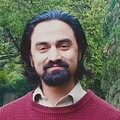
The version of Java installed with Android Studio on my Mac is the following (when I run java -version)
openjdk version "1.8.0_242-relea...
New
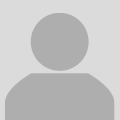
Hi everyone. Getting right to my question, I have recently thought about implementing payment by installment feature in my website. Who k...
New
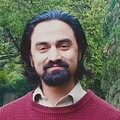
I have always used antique keyboards like Cherry MX 1800 or Cherry MX 8100 and almost always have modified the switches in some way, like...
New
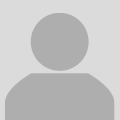
I have an array of strings in JavaScript, and I need to convert it into a single string with specific delimiter characters between the el...
New
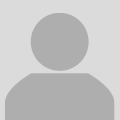
I’m working on a web application where users can sign up with their email addresses. To ensure data integrity, I want to implement client...
New
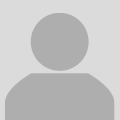
Hi, I’m now investigating the complexities of Python loops, specifically the contrast between for and while loops. However, I’ve had some...
New
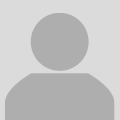
Hello,
I am new to this forum. Not really sure if this topic is relevant for this chat at all. I apologize if its not.
I am trying to c...
New
Other popular topics
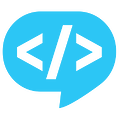
Hello Devtalk World!
Please let us know a little about who you are and where you’re from :nerd_face:
New
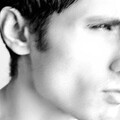
A thread that every forum needs!
Simply post a link to a track on YouTube (or SoundCloud or Vimeo amongst others!) on a separate line an...
New
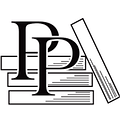
A PragProg Hero’s Journey with Brian P. Hogan @bphogan
Have you ever worried that your only legacy will be in the form of legacy...
New
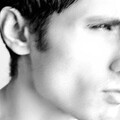
SpaceVim seems to be gaining in features and popularity and I just wondered how it compares with SpaceMacs in 2020 - anyone have any thou...
New
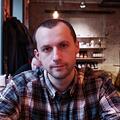
Intensively researching Erlang books and additional resources on it, I have found that the topic of using Regular Expressions is either c...
New
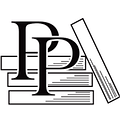
Rails 7 completely redefines what it means to produce fantastic user experiences and provides a way to achieve all the benefits of single...
New
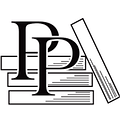
Build efficient applications that exploit the unique benefits of a pure functional language, learning from an engineer who uses Haskell t...
New
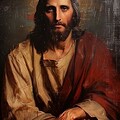
The File System Access API with Origin Private File System.
WebKit supports new API that makes it possible for web apps to create, open,...
New
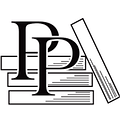
Author Spotlight:
David Bryant Copeland
@davetron5000
We’re so happy to bring you another Author Spotlight, a series where we sit dow...
New
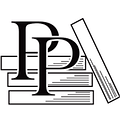
A Ruby-Centric Chat with Noel Rappin @noelrappin
Once you start noodling around with Ruby you quickly figure out, as Noel Rappi...
New
Categories:
Sub Categories:
- All
- In The News (10019)
- Dev Chat (200)
- Questions
- Resources (118)
- Blogs/Talks (26)
- Jobs (3)
- Events (15)
- Code Editors (58)
- Hardware (57)
- Reviews (4)
- Sales (15)
- Design & UX (4)
- Marketing & SEO (1)
- Industry & Culture (14)
- Ethics & Privacy (19)
- Business (4)
- Learning Methods (4)
- Content Creators (7)
- DevOps & Hosting (9)
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /emacs
- /haskell
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /tailwind
- /kotlin
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /textmate
- /debian
- /sublime-text
- /nixos
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /ubuntu
- /manjaro
- /django
- /spring
- /diversity
- /lua
- /nodejs
- /slackware
- /julia
- /c
- /neovim