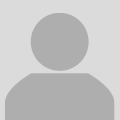
harwind
Java's for each loop
So, basically, I’m writing a method to check application numbers in my array ‘applications,’ and this method receives a parameter to verify that specific application number and compares it to the application numbers in the list.
So first look at the code:
public boolean hasApplicationNumber(int number) {
if (number <= 3 ) {
throw new IllegalArgumentException("bad number");
}
for (ApplicationData current : applications) {
if (number == current.getApplicationNumber() ) {
return true;
}
}
return false;
}
JUnit test to validate the application number:
public void shouldHaveApplicationNumber1() {
University westGeorgia = new University("West Georgia");
ApplicationData student1 = new ApplicationData(9, 7.0, 400);
westGeorgia.addApplication(student1);
assertEquals(true, westGeorgia.hasApplicationNumber(1));
}
This JUnit test is failing because my code returns “false” instead of “true.”
However, if I change the return values in my “hasApplicationNumber” method to true (at the very bottom of the method), this test will pass, but another test I have (that doesn’t allow the list to exceed 10) will return “true” when it is supposed to be “false,” causing that test to fail (I didn’t include that test because it is very similar to the one I have already provided — just “1” is changed to “10” and “true”
I’m beginning to suspect that either my test is being ignored by the Java compiler, or my for-each loop is executing correctly and returning “true,” but since I followed this documentation, I have that lingering false at the very end, which may be declaring the item false nonetheless.
I might be overthinking this, but I’m at a loss on how to change the for-each loop in the method I wrote. Any assistance in sorting this out would be much appreciated!
Most Liked
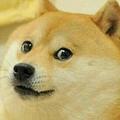
dtonhofer
This JUnit test is failing because my code returns “false” instead of “true.”
Isn’t the JUnit test failing because the
hasApplicationNumber(1)
throws?
At this point, the easiest way to unconfuse yourself is to add a few print statements into the test code.
System.out.println("After test for throw );
etc.
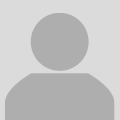
finner
hi @harwind -
According to the code you have pasted when you pass 1
into the method hasApplicationNumber
an IllegalArgumentException
will be thrown. So the test should fail.
I’ve also noticed that you are using double
in the ApplicationData
class but treating them as int
in the hasApplicationNumber
method.
Here is a stripped down version without the ApplicationData
class and using int
.
public class DevTalkTest {
private final int[] numbers = new int[]{9, 7, 400};
@Test
public void shouldHaveApplicationNumber1() {
assertTrue(hasApplicationNumber(1));
}
public boolean hasApplicationNumber(int number) {
if (number <= 3) {
throw new IllegalArgumentException("bad number");
}
for (int num : numbers) {
if (num == number) return true;
}
return false;
}
}
The tests will fail with the error:
bad number
java.lang.IllegalArgumentException: bad number
. . .
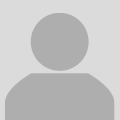
finner
I was going to suggest setting a breakpoint on the last line.
Popular General Dev topics
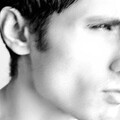
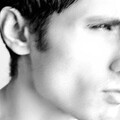
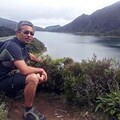
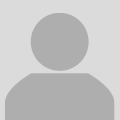
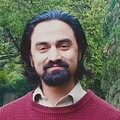
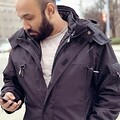
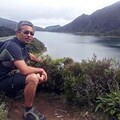
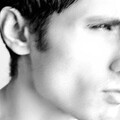
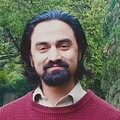
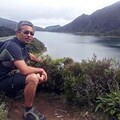
Other popular topics
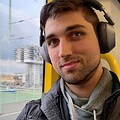
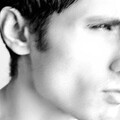
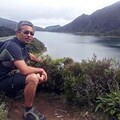
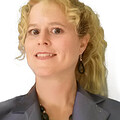
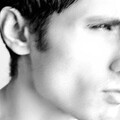
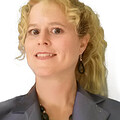
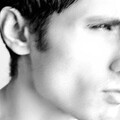
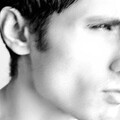
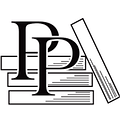
Categories:
Sub Categories:
- All
- In The News (10039)
- Dev Chat
- Questions (32)
- Resources (118)
- Blogs/Talks (26)
- Jobs (3)
- Events (15)
- Code Editors (58)
- Hardware (57)
- Reviews (4)
- Sales (15)
- Design & UX (4)
- Marketing & SEO (1)
- Industry & Culture (14)
- Ethics & Privacy (19)
- Business (4)
- Learning Methods (4)
- Content Creators (7)
- DevOps & Hosting (9)
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /emacs
- /haskell
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /debian
- /sublime-text
- /textmate
- /nixos
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /ubuntu
- /spring
- /django
- /manjaro
- /diversity
- /nodejs
- /lua
- /slackware
- /c
- /julia
- /markdown