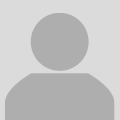
harwind
C++ String to Integer Conversion
I’m working on a C++ program where I need to convert a string containing a numeric value into an integer. I want to ensure that this conversion is handled correctly and safely, especially when dealing with potential exceptions or invalid input.
Here’s a simplified example of what I’m trying to do:
#include <iostream>
#include <string>
int main() {
std::string str = "12345"; // This could be any numeric string.
// How can I safely convert the string 'str' to an integer?
int num = ???; // The converted integer should be stored here.
std::cout << "Converted integer: " << num << std::endl;
return 0;
}
In this code, I have a string str
containing a numeric value. I want to convert this string into an integer variable num
. However, I want to handle potential issues gracefully, such as cases where the string is not a valid integer. Could you offer a C++ code sample illustrating the proper and secure approach to convert a string to an integer while managing any potential exceptions or errors? I appreciate you helping me. I attempted to visit multiple sites like Scaler to locate the answer, but I was unable to do so. Thank you.
Most Liked
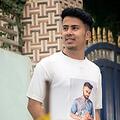
gulshan212
Well, I can see some logical issues with your code.
Can you try this code and confirm wether it is working or not.
#include <iostream>
#include <string>
#include <stdexcept> // Include this for std::invalid_argument and std::out_of_range
int main() {
std::string str = "12345"; // This could be any numeric string.
int num = 0; // The converted integer will be stored here.
try {
num = std::stoi(str);
std::cout << "Converted integer: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Invalid argument: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "Out of range: " << e.what() << std::endl;
}
return 0;
}
Thanks
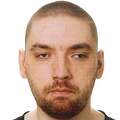
Eiji
I’m not a senior C++
developer, so I have no idea about the best way, but this should work:
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "123";
// str[0] - gets the first character
// isdigit(character) - checks if character is a digit
// stoi throws invalid_argument exception
// if string does not start with a digit
if (isdigit(str[0])) {
/*
stoi means "String TO Integer"
you can easily change it to for example:
* stof ("String TO Float"),
* stol ("String TO Long")
* and so on …
*/
int num = std::stoi(str);
std::cout << "[DONE] Converted integer: " << num << std::endl;
} else {
std::cout << "[ERROR] Invalid input string: " << str << std::endl;
}
return 0;
}
Helpful resources
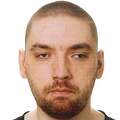
Eiji
For me looks good. I have tested it on my MX Linux
distribution.
It’s interesting how much the code says about it’s author. In my case 7 years in Elixir
gives result …
-
Even if somehow I remember somewhat about
try/catch
I was still looking for a “better” solution, so I have added one extra dependency for a singleisdigit
call. -
std:err
of course! When I saw your code I have reminded it immediately. -
stdexcept
is interesting. Maybe I have used it, but I don’t remember it much especiallye.what()
call. I guess that I haven’t used multiple catch blocks before inC++
. -
out_of_range
error handling is simple, but also brilliant idea. I’m not surprised that I wasn’t thinking about such edge case since inElixir
we have justInteger
for all well … integers (no small or big ones). -
I only wonder why you define
num
variable outside oftry
block having in mind it’s only usage is within it, but that’s definitely not the most important thing.
So much to learn in such a small code, thanks!
Adding additional links below to the documentation pages:
Popular General Dev topics
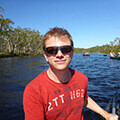
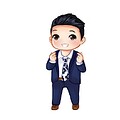
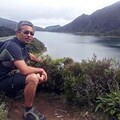
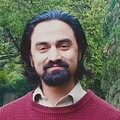
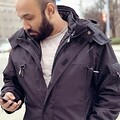
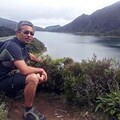
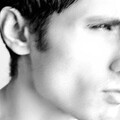
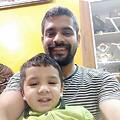
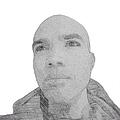
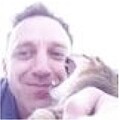
Other popular topics
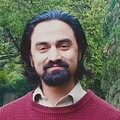
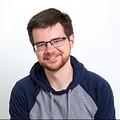
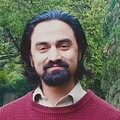
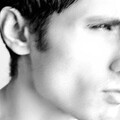
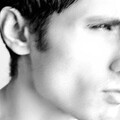
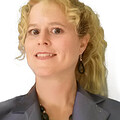
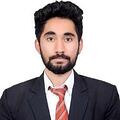
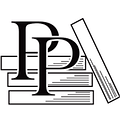
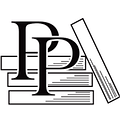
Categories:
Sub Categories:
- All
- In The News (10036)
- Dev Chat
- Questions (32)
- Resources (118)
- Blogs/Talks (26)
- Jobs (3)
- Events (15)
- Code Editors (58)
- Hardware (57)
- Reviews (4)
- Sales (15)
- Design & UX (4)
- Marketing & SEO (1)
- Industry & Culture (14)
- Ethics & Privacy (19)
- Business (4)
- Learning Methods (4)
- Content Creators (7)
- DevOps & Hosting (9)
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /emacs
- /haskell
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /scala
- /zig
- /sublime-text
- /debian
- /textmate
- /nixos
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /ubuntu
- /manjaro
- /django
- /spring
- /diversity
- /nodejs
- /lua
- /slackware
- /c
- /julia
- /neovim