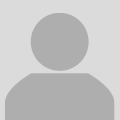
sona11
In Java, convert a string array to a jagged array
I have a 1D array of numbers and need help splitting them into groups using a jagged array so that I can perform a series of computations. e.g.
int array []={1 2 3 4 5 6 7 8 9 10}
I want to segregate and allocate the first four to one array, the next three to another, and the final three to a third. In my code, I was expecting to obtain something like this.
int [][] x = new int [3][];
x[0] = new int [4];
x[0] = {1 2 3 4};
x[1] = new int [3];
x[1] = {5 6 7};
x[2] = new int [3];
x[2] = {8 9 10};
Is there any method to create this jagged array using a flexible for loop and divide it into M groups or N groups of N values each just like an example given here that I didn’t understand? I attempted to retrieve those numbers using substring(), but I’m not sure how. I attempted to get those numbers using substring(), but I’m not sure how to proceed or if I’m doing it correctly.
for( int i=0; i<x.length; i++) {
x [i]= array.substring (0,3);
x [i]=array.substring (4,6);
x [i]=array.substring(7,9);
}
I’m new to programming, but this code is plainly incorrect; could you kindly assist me? Thank you very much.
Most Liked
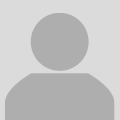
finner
hi @sona11 - this is a very good problem for learning how to program.
There is no method in Java to do this, you need to write it. I want to help you but I am not going to give you the code, you will not learn that way.
int array []={1 2 3 4 5 6 7 8 9 10}
Your example array is an array of int
which is a primitive data type in Java, not an object like String
, List
, etc.
The substring()
in Java is used with the String
object which is why it will not work with an array or an int
.
Examples:
String s = "Jagged Array";
s.substring(7); // will return Array
s.substring(0, 6); // will return Jagged
The example you refer to can help.
Copy that example to your IDE and change the following lines:
Replace this;
Scanner scn = new Scanner(System.in);
//Taking the input from user
int r = scn.nextInt();
with this:
int r = 3;
We are just removing the user input from the code and hardcoding the number of rows, in this case we put 3
.
Then run it in debug. You can also change the value of r
each time you run to see what happens. This should help you understand what it is doing.
Pay special attention to the nested loop when you are stepping in debug.
int temp = 0;
for (int i = 0; i < arr.length; i++)
for (int j = 0; j < arr[i].length; j++)
arr[i][j] = temp++;
Once you understand that example you can start thinking about how to make it more flexible. I will try to help you with that if you want (but I won’t give you code).
What version of Java are you using? And what IDE, if you are using an IDE?
I hope this helps you in the learning process.
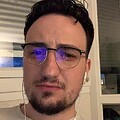
Maartz
Hi,
Well to achieve some sort of output,
I’d use a regex with a capture. By explicitly saying I want a 4/3/3 capture.
The Regex should look like this:
[0-9]{4}\b-[0-9]{3}\b-[0-9]{3}
Note that this regex expects a ‘-’ as separator. Making use whitespace should do the trick.
What do you think about it?
Popular Frontend topics
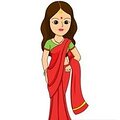
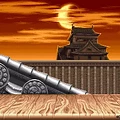
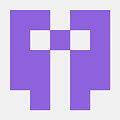
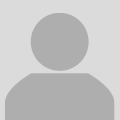
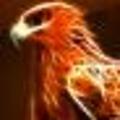
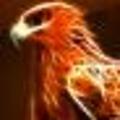
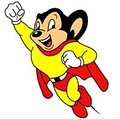
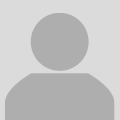
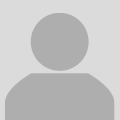
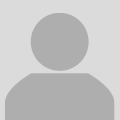
Other popular topics
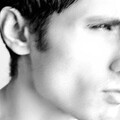
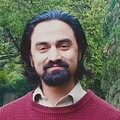
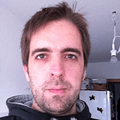
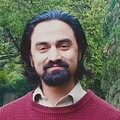
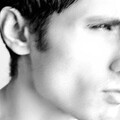
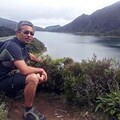
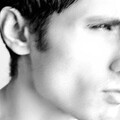
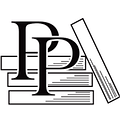
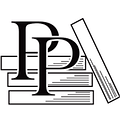
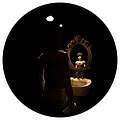
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /emacs
- /haskell
- /java
- /onivim
- /svelte
- /typescript
- /crystal
- /kotlin
- /c-plus-plus
- /tailwind
- /gleam
- /ocaml
- /react
- /elm
- /flutter
- /vscode
- /ash
- /opensuse
- /centos
- /html
- /php
- /deepseek
- /zig
- /scala
- /lisp
- /textmate
- /sublime-text
- /debian
- /nixos
- /react-native
- /agda
- /kubuntu
- /arch-linux
- /revery
- /django
- /ubuntu
- /spring
- /manjaro
- /diversity
- /nodejs
- /lua
- /c
- /slackware
- /julia
- /markdown