Functional Programming in Java, Second Edition: p.65 "WatchFileChange.java"
Suggesting a text change:
We read:
We’ve registered a WatchService to observe any change to the current directory.
but what actually happens is
We’ve registered a path with a filesystem
WatchService
to get told about ‘modification’ changes to the current directory.
On my system, file deletion does not give rise to a notification, but creation does (probably because after creation, the file is additionally modified)
The text
"Report any file changed within next 1 minute..."
should really say
System.out.println("Report the first change on '" + path + "' within the next 1 minute...");
Note that the code given uses an inner loop. At this point, I really feel we should use collect()
:
if(watchKey != null) {
watchKey.pollEvents()
.stream()
.forEach(event ->
System.out.println(event.context()));
}
Nicer:
String res = (watchKey == null) ? "nothing happened at all!" :
watchKey.pollEvents()
.stream()
.map(event -> event.context().toString())
.collect(Collectors.joining(", "));
As I had some trouble understanding how the WatchService actually works and what those keys are doing, here is the full method
public void watchFileChange() throws IOException {
final Path path = Paths.get(theDir);
String res;
// Try-with-resources to close the WatchService at the end
// (and thus cancel all the WatchKeys registered with it)
try (WatchService watchService = path.getFileSystem().newWatchService()) {
try {
// No need to retain the WatchKey returned by path.register()
path.register(watchService, StandardWatchEventKinds.ENTRY_MODIFY);
System.out.println("Report the first change on '" + path + "' within the next 1 minute...");
WatchKey watchKey = null;
// poll() "Retrieves and removes the next watch key, waiting if necessary up to
// the specified wait time if none are yet present."
try {
watchKey = watchService.poll(1, TimeUnit.MINUTES);
} catch (InterruptedException ex) {
System.out.println("Got interrupted");
}
res = (watchKey == null) ? "nothing happened at all!" :
watchKey.pollEvents()
.stream()
.map(event -> event.context().toString())
.collect(Collectors.joining(", "));
} catch (NoSuchFileException ex) {
res = "Looks like there is no filesystem entry '" + path + "'";
}
}
System.out.println(res);
}
Popular Prag Prog topics
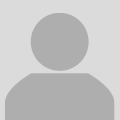
Whilst the author has been careful to provide exact results for the tests elsewhere in the book (such as surds with the transformation te...
New
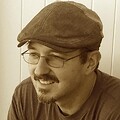
The following is cross-posted from the original Ray Tracer Challenge forum, from a post by garfieldnate. I’m cross-posting it so that the...
New
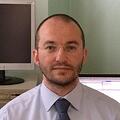
Hi Jamis,
I think there’s an issue with a test on chapter 6. I own the ebook, version P1.0 Feb. 2019.
This test doesn’t pass for me:
...
New
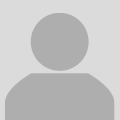
Hello! On page xix of the preface, it says there is a community forum "… for help if your’re stuck on one of the exercises in this b...
New
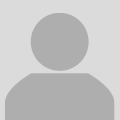
This isn’t directly about the book contents so maybe not the right forum…but in some of the code apps (e.g. turbo/06) it sends a TURBO_ST...
New
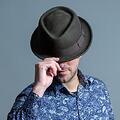
In case this helps anyone, I’ve had issues setting up the rails source code. Here were the solutions:
In Gemfile, change
gem 'rails...
New
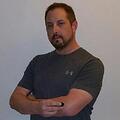
I’m under the impression that when the reader gets to page 136 (“View Data with the Database Inspector”), the code SHOULD be able to buil...
New
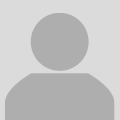
Is the book’s epub format available to read on Google Play Books?
New
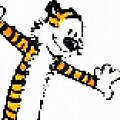
Modern Front-End Development for Rails - application does not start after run bin/setup (page xviii)
After some hassle, I was able to finally run bin/setup, now I have started the rails server but I get this error message right when I vis...
New
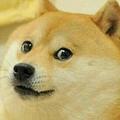
@parrt
In the context of Chapter 4.3, the grammar Java.g4, meant to parse Java 6 compilation units, no longer passes ANTLR (currently 4....
New
Other popular topics
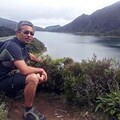
I am thinking in building or buy a desktop computer for programing, both professionally and on my free time, and my choice of OS is Linux...
New
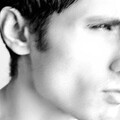
Curious to know which languages and frameworks you’re all thinking about learning next :upside_down_face:
Perhaps if there’s enough peop...
New
New
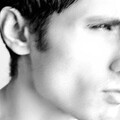
Do the test and post your score :nerd_face:
:keyboard:
If possible, please add info such as the keyboard you’re using, the layout (Qw...
New
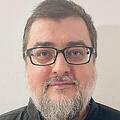
Small essay with thoughts on macOS vs. Linux:
I know @Exadra37 is just waiting around the corner to scream at me “I TOLD YOU SO!!!” but I...
New
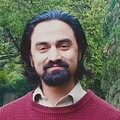
The V Programming Language
Simple language for building maintainable programs
V is already mentioned couple of times in the forum, but I...
New
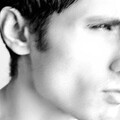
Saw this on TikTok of all places! :lol:
Anyone heard of them before?
Lite:
New
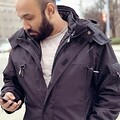
This is going to be a long an frequently posted thread.
While talking to a friend of mine who has taken data structure and algorithm cou...
New
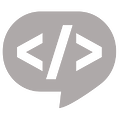
The overengineered Solution to my Pigeon Problem.
TL;DR: I built a wifi-equipped water gun to shoot the pigeons on my balcony, controlle...
New
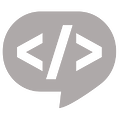
Large Language Models like ChatGPT say The Darnedest Things.
The Errors They MakeWhy We Need to Document Them, and What We Have Decided ...
New
Latest in PragProg
Latest (all)
Categories:
My Saved Portals
-
None saved yet
Popular Portals
- /elixir
- /opensuse
- /rust
- /kotlin
- /ruby
- /erlang
- /python
- /clojure
- /react
- /quarkus
- /go
- /vapor
- /v
- /react-native
- /wasm
- /security
- /django
- /nodejs
- /centos
- /haskell
- /rails
- /fable
- /gleam
- /swift
- /js
- /deno
- /assemblyscript
- /tailwind
- /laravel
- /symfony
- /phoenix
- /crystal
- /typescript
- /debian
- /adonisjs
- /julia
- /arch-linux
- /svelte
- /spring
- /preact
- /flutter
- /c-plus-plus
- /actix
- /java
- /angular
- /ocaml
- /zig
- /kubuntu
- /scala
- /zotonic
- /vim
- /rocky
- /lisp
- /html
- /keyboards
- /vuejs
- /nim
- /emacs
- /nerves
- /elm