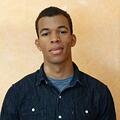
ryanzidago
Genetic Algorithms in Elixir: Wrong function to calculate the average genes (page 148)
The average_tiger
function is written like so in the book:
def average_tiger(population) do
genes = Enum.map(population, & &1.genes)
fitnesses = Enum.map(population, & &1.fitness)
ages = Enum.map(population, & &1.age)
num_tigers = length(population)
avg_fitness = Enum.sum(fitnesses) / num_tigers
avg_age = Enum.sum(ages) / num_tigers
avg_genes =
genes
|> Enum.zip()
|> Enum.map(&(Enum.sum(&1) / num_tigers))
%Chromosome{genes: avg_genes, age: avg_age, fitness: avg_fitness}
end
Genes is a list of list:
[
[1, 1, 1, 0, 1, 1, 0, 1],
[0, 1, 1, 1, 1, 0, 1, 0],
[1, 1, 1, 0, 0, 0, 0, 0],
[0, 0, 1, 1, 1, 1, 0, 0],
[0, 1, 0, 1, 1, 0, 0, 0],
[1, 1, 0, 0, 1, 1, 0, 1],
[0, 1, 0, 1, 0, 0, 0, 1],
[1, 0, 1, 1, 0, 1, 0, 0],
[0, 0, 1, 1, 0, 1, 0, 0],
[0, 1, 0, 0, 1, 0, 0, 1],
[0, 1, 0, 1, 1, 0, 1, 1],
[0, 0, 1, 1, 0, 1, 1, 1],
[0, 0, 1, 1, 0, 0, 1, 1],
[1, 1, 0, 0, 0, 0, 1, 1],
[0, 0, 0, 1, 0, 1, 0, 0],
[0, 0, 0, 1, 1, 0, 0, 0],
[1, 0, 0, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 1, 0, 1],
[1, 0, 0, 1, 1, 0, 1, 1],
[0, 0, 0, 0, 1, 0, 0, 0]
]
With Enum.zip/1
this list of lists is transformed to a list of tuples (with columns zipped together):
[
{1, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0},
{1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0},
{1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0},
{1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1},
{1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0},
{0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0},
{1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0}
]
The problem is that Tuple
does not implement the Enumerable
protocol so it is not possible to map the element of tuple like this:
genes
|> Enum.zip()
|> Enum.map(&(Enum.sum(&1) / num_tigers))
I think some code got los in the process
To calculate the average genes, the code should look like this:
avg_genes =
genes
|> Enum.zip()
|> Enum.map(&Tuple.to_list/1)
|> Enum.map(&Enum.sum/1)
|> Enum.map(&(&1 / num_tigers))
|> Enum.map(&Float.round(&1, 2))
Popular Pragmatic Bookshelf topics
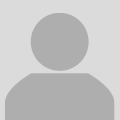
Hello Brian,
I have some problems with running the code in your book. I like the style of the book very much and I have learnt a lot as...
New
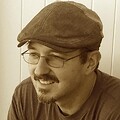
The following is cross-posted from the original Ray Tracer Challenge forum, from a post by garfieldnate. I’m cross-posting it so that the...
New
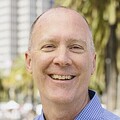
I thought that there might be interest in using the book with Rails 6.1 and Ruby 2.7.2. I’ll note what I needed to do differently here.
...
New
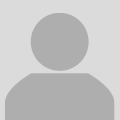
Hi,
build fails on:
bracket-lib = “~0.8.1”
when running on Mac Mini M1 Rust version 1.5.0:
Compiling winit v0.22.2
error[E0308]: mi...
New
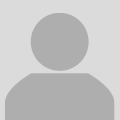
Is the book’s epub format available to read on Google Play Books?
New
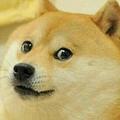
@parrt
In the context of Chapter 4.3, the grammar Java.g4, meant to parse Java 6 compilation units, no longer passes ANTLR (currently 4....
New
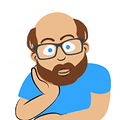
Hello @herbert ! Trying to get the very first “Hello, Bracket Terminal!" example to run (p. 53). I develop on an Amazon EC2 instance runn...
New
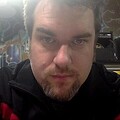
Docker-Machine became part of the Docker Toolbox, which was deprecated in 2020, long after Docker Desktop supported Docker Engine nativel...
New
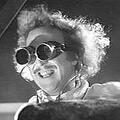
Getting an error when installing the dependencies at the start of this chapter:
could not compile dependency :exla, "mix compile" failed...
New
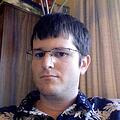
I’ve got to the end of Ch. 11, and the app runs, with all tabs displaying what they should – at first. After switching around between St...
New
Other popular topics
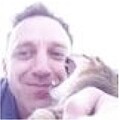
Any thoughts on Svelte?
Svelte is a radical new approach to building user interfaces. Whereas traditional frameworks like React and Vue...
New
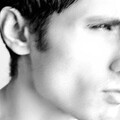
You might be thinking we should just ask who’s not using VSCode :joy: however there are some new additions in the space that might give V...
New
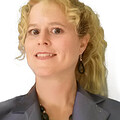
Hello content creators! Happy new year. What tech topics do you think will be the focus of 2021? My vote for one topic is ethics in tech...
New
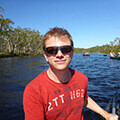
Not sure if following fits exactly this thread, or if we should have a hobby thread…
For many years I’m designing and building model air...
New
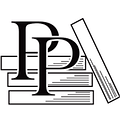
Learn different ways of writing concurrent code in Elixir and increase your application's performance, without sacrificing scalability or...
New
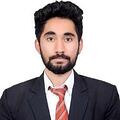
API 4
Path:
/user/following/
Method:
GET
Description:
Returns the list of all names of people whom the user follows
Response
[
{ ...
New
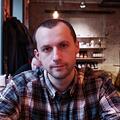
Intensively researching Erlang books and additional resources on it, I have found that the topic of using Regular Expressions is either c...
New
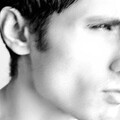
We’ve talked about his book briefly here but it is quickly becoming obsolete - so he’s decided to create a series of 7 podcasts, the firs...
New
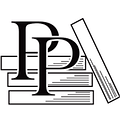
Rails 7 completely redefines what it means to produce fantastic user experiences and provides a way to achieve all the benefits of single...
New
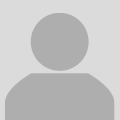
Hello,
I’m a beginner in Android development and I’m facing an issue with my project setup. In my build.gradle.kts file, I have the foll...
New
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /ruby
- /wasm
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /emacs
- /haskell
- /java
- /onivim
- /svelte
- /typescript
- /crystal
- /kotlin
- /c-plus-plus
- /tailwind
- /gleam
- /ocaml
- /react
- /flutter
- /elm
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /sublime-text
- /textmate
- /lisp
- /debian
- /nixos
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /django
- /ubuntu
- /manjaro
- /spring
- /diversity
- /nodejs
- /lua
- /slackware
- /c
- /julia
- /markdown