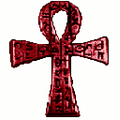
Red
Rust Brain Teasers: Comments on puzzle 19/async (page 82-)
An open discussion about this particular puzzle on asynchronous code, since I’m only learning and I may have overlooked something.
1.) About tokio
, you either have to declare the main as
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> { ... }
or if main is something else, it also possible to use its own block_on
(or other alternative) to launch the asynchronous code. In this case, you have to enable the time “reactor” to avoid a panic:
fn main() {
let rt = runtime::Builder::new_multi_thread()
.enable_time()
.build()
.unwrap();
rt.block_on(test()).unwrap();
}
with, for example
async fn test() -> Result<(), Box<dyn std::error::Error>> {
join!(count_and_wait(1), count_and_wait(2), count_and_wait(3));
Ok(())
}
or directly inserting an async block: rt.block_on(async { ... })
.
2.) There is another alternative to using tokio::time::sleep
or a thread-oriented approach.
The problem comes from using a thread
function, which is not meant for this sort of asynchronous pattern as very well explained pages 82 and 85. We actually get the same issue in other languages like Kotlin. There is no .await
and so no real “break” that will allow to switch to another async code.
There is an asynchronous async_std::task::sleep
function which supports .await
and will give the expected outcome:
use async_std::task;
async fn count_and_wait(n: u64) -> u64 {
println!("starting {}", n);
task::sleep(Duration::from_millis(n * 100)).await;
println!("returning {}", n);
n
}
Popular Pragmatic Bookshelf topics
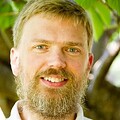
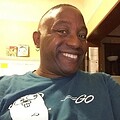
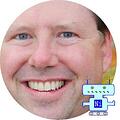
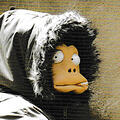
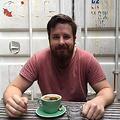
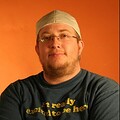
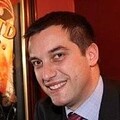
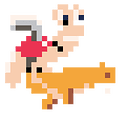
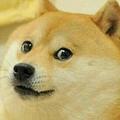
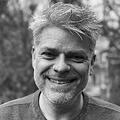
Other popular topics
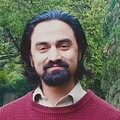
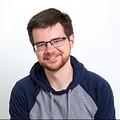
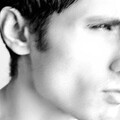
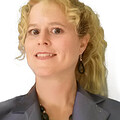
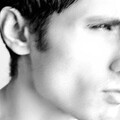
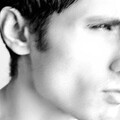
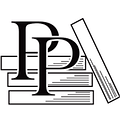
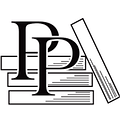
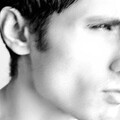
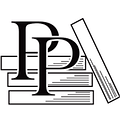
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /wasm
- /ruby
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /java
- /emacs
- /haskell
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /react
- /flutter
- /elm
- /ocaml
- /ash
- /vscode
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /zig
- /scala
- /textmate
- /debian
- /sublime-text
- /nixos
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /revery
- /ubuntu
- /django
- /manjaro
- /spring
- /diversity
- /nodejs
- /lua
- /slackware
- /c
- /julia
- /markdown