Enum: Behind the scenes
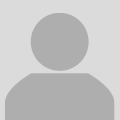
I’ve never really felt 100% comfortable using the enum
type because of my lack of understanding how it is constructed . . .
. . . until now
Here is how a basic enum
might be typically declared.
enum DevTalkForum {
NEWS,
CHAT,
JOURNALS,
BOOK_ERRATA
}
And here is the class that is constructed behinds the scenes:
final class DevTalkForum extends Enum<DevTalkForum> {
/**
* the type Enum only has 2 simple properties:
*/
private DevTalkForum(String name, int ordinal) {
super(name, ordinal);
}
/**
* each element in the enum is a subclass of the type Enum
*/
public static final DevTalkForum NEWS = new DevTalkForum("NEWS", 0);
public static final DevTalkForum CHAT = new DevTalkForum("CHAT", 1);
public static final DevTalkForum JOURNALS = new DevTalkForum("JOURNALS", 2);
public static final DevTalkForum BOOK_ERRATA = new DevTalkForum("BOOK_ERRATA", 3);
/**
* all the enum classes are stored in an array
*/
private static final DevTalkForum[] VALUES = { NEWS, CHAT, JOURNALS, BOOK_ERRATA };
public static DevTalkForum[] values() {
return VALUES.clone();
}
public static DevTalkForum valueOf(String name) {
for (DevTalkForum e : VALUES)
if (e.name().equals(name)) return e;
throw new IllegalArgumentException();
}
}
Realising how the enum
is constructed has helped me understand how to use it correctly.
For example, the toString()
of the class can be overridden to provide an alternative string value if you do not want to add another property.
enum DevTalkForum {
NEWS,
CHAT,
JOURNALS,
BOOK_ERRATA;
@Override
public String toString() {
switch (this) {
case CHAT:
return "Chat";
case JOURNALS:
return "Journals";
case BOOK_ERRATA:
return "Book Errata";
case NEWS:
default:
return "News";
}
}
}
So now that I feel closer to the enum
I think I’m going to try and pop them into my code a bit more often.
Hope this was somehow helpful
Popular Backend topics
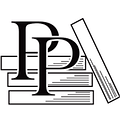
Real-time applications come with real challenges—persistent connections, multi-server deployment, and strict performance requirements are...
New
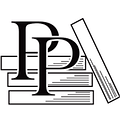
Don’t accept the compromise between fast and beautiful: you can have it all. Phoenix creator Chris McCord, Elixir creator José Valim, and...
New
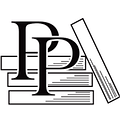
Your domain is rich and interconnected, and your API should be, too. Upgrade your web API to GraphQL, using flexible queries to empower y...
New
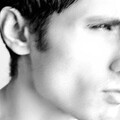
Currently a hot topic in the BEAM world, let’s start a thread for it (as suggested by @crowdhailer here) :smiley:
What are your current...
New
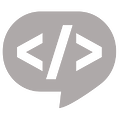
Node.js v12.20.0, v15.3.0 and v14.15.1 has been released.
Link: Release 2020-11-24, Version 12.20.0 'Erbium' (LTS), @mylesborin...
New
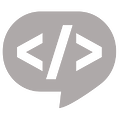
Ruby on Rails v6.1.0 has been released.
Link: Release 6.1.0 · rails/rails · GitHub
New
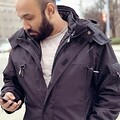
A friend and I implemented a few algorithms in Dart and set them up in a repository. Thought I’d share it here.
New
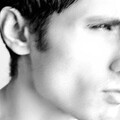
In case anyone else is wondering why Ruby 3 doesn’t show when you do asdf list-all ruby :man_facepalming: do this first:
asdf plugin-upd...
New
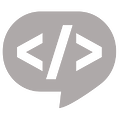
A new PostgreSQL blog post/announcement has been posted!
Get the full details here: PostgreSQL: Generate realistic test Data for Postgr...
New
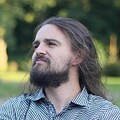
I have being some Elixir open-source contributions and side projects. Oh, and I’m doing them on livestreams on my twitch channel, follow ...
New
Other popular topics
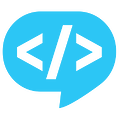
Hello Devtalk World!
Please let us know a little about who you are and where you’re from :nerd_face:
New
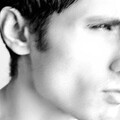
A thread that every forum needs!
Simply post a link to a track on YouTube (or SoundCloud or Vimeo amongst others!) on a separate line an...
New
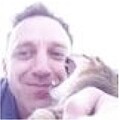
Any thoughts on Svelte?
Svelte is a radical new approach to building user interfaces. Whereas traditional frameworks like React and Vue...
New
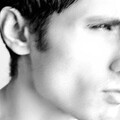
Just done a fresh install of macOS Big Sur and on installing Erlang I am getting:
asdf install erlang 23.1.2
Configure failed.
checking ...
New
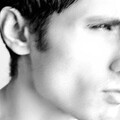
Do the test and post your score :nerd_face:
:keyboard:
If possible, please add info such as the keyboard you’re using, the layout (Qw...
New
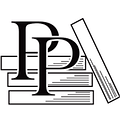
A Hero’s Journey with Chris Pine
Chris Pine, author of Learn to Program, Third Edition, discusses his journey to becoming a Pragm...
New
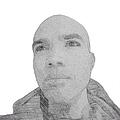
A few weeks ago I started using Warp a terminal written in rust. Though in it’s current state of development there are a few caveats (tab...
New
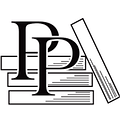
Author Spotlight: James Stanier (@jstanier)
James Stanier, author of Effective Remote Work , discusses how to rethink the office as we...
New
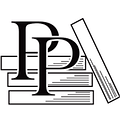
Author Spotlight: David Bryant Copeland (@davetron5000)
We’re so happy to bring you another Author Spotlight, a series where we sit d...
New
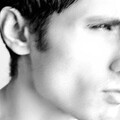
This is cool!
DEEPSEEK-V3 ON M4 MAC: BLAZING FAST INFERENCE ON APPLE SILICON
We just witnessed something incredible: the largest open-s...
New
Latest in Backend
Latest (all)
Categories:
My Saved Portals
-
None saved yet
Popular Portals
- /elixir
- /opensuse
- /rust
- /kotlin
- /ruby
- /erlang
- /python
- /clojure
- /react
- /quarkus
- /go
- /vapor
- /v
- /react-native
- /wasm
- /security
- /django
- /nodejs
- /centos
- /haskell
- /rails
- /fable
- /gleam
- /swift
- /js
- /deno
- /assemblyscript
- /tailwind
- /laravel
- /symfony
- /phoenix
- /crystal
- /typescript
- /debian
- /adonisjs
- /julia
- /arch-linux
- /svelte
- /spring
- /c-plus-plus
- /preact
- /flutter
- /actix
- /java
- /angular
- /ocaml
- /zig
- /kubuntu
- /scala
- /zotonic
- /vim
- /rocky
- /lisp
- /html
- /keyboards
- /vuejs
- /nim
- /emacs
- /nerves
- /elm