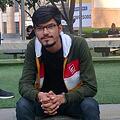
lochanchugh
Microphone input in website in webview for swiftui
I have a website of a chatbot that also accepts microphone inputs and I embedded that into my swift and the everything other than the mic is working. I have included permission in the info file and here’s some of the code :
import SwiftUI
import WebKit
import AVFoundation
struct WebView: UIViewRepresentable {
let url: URL
class Coordinator: NSObject, WKUIDelegate, WKNavigationDelegate {
var parent: WebView
init(parent: WebView) {
self.parent = parent
}
func webView(_ webView: WKWebView, decidePolicyFor navigationAction: WKNavigationAction, decisionHandler: @escaping (WKNavigationActionPolicy) -> Void) {
decisionHandler(.allow)
}
func webView(_ webView: WKWebView, runJavaScriptAlertPanelWithMessage message: String, initiatedByFrame frame: WKFrameInfo, completionHandler: @escaping () -> Void) {
let alert = UIAlertController(title: nil, message: message, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: { _ in
completionHandler()
}))
parent.presentAlert(alert: alert)
}
func webView(_ webView: WKWebView, runJavaScriptConfirmPanelWithMessage message: String, initiatedByFrame frame: WKFrameInfo, completionHandler: @escaping (Bool) -> Void) {
let alert = UIAlertController(title: nil, message: message, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: { _ in
completionHandler(true)
}))
alert.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: { _ in
completionHandler(false)
}))
parent.presentAlert(alert: alert)
}
func webView(_ webView: WKWebView, runJavaScriptTextInputPanelWithPrompt prompt: String, defaultText: String?, initiatedByFrame frame: WKFrameInfo, completionHandler: @escaping (String?) -> Void) {
let alert = UIAlertController(title: prompt, message: nil, preferredStyle: .alert)
alert.addTextField { textField in
textField.text = defaultText
}
alert.addAction(UIAlertAction(title: "OK", style: .default, handler: { _ in
completionHandler(alert.textFields?.first?.text)
}))
alert.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: { _ in
completionHandler(nil)
}))
parent.presentAlert(alert: alert)
}
func webView(_ webView: WKWebView, requestMediaCapturePermissionFor origin: WKSecurityOrigin, initiatedByFrame frame: WKFrameInfo, type: WKMediaCaptureType, decisionHandler: @escaping (WKPermissionDecision) -> Void) {
decisionHandler(.grant)
}
func webView(_ webView: WKWebView, didFail navigation: WKNavigation!, withError error: Error) {
print("Navigation error: \(error.localizedDescription)")
}
}
func makeCoordinator() -> Coordinator {
Coordinator(parent: self)
}
func makeUIView(context: Context) -> WKWebView {
let webConfiguration = WKWebViewConfiguration()
webConfiguration.allowsInlineMediaPlayback = true
webConfiguration.mediaTypesRequiringUserActionForPlayback = []
let webView = WKWebView(frame: .zero, configuration: webConfiguration)
webView.uiDelegate = context.coordinator
webView.navigationDelegate = context.coordinator
requestMicrophoneAccess()
return webView
}
func updateUIView(_ webView: WKWebView, context: Context) {
let request = URLRequest(url: url)
webView.load(request)
}
func requestMicrophoneAccess() {
AVAudioSession.sharedInstance().requestRecordPermission { granted in
DispatchQueue.main.async {
if granted {
print("Microphone access granted")
} else {
print("Microphone access denied")
}
}
}
}
func presentAlert(alert: UIAlertController) {
if let windowScene = UIApplication.shared.connectedScenes.first as? UIWindowScene,
let rootViewController = windowScene.windows.first?.rootViewController {
rootViewController.present(alert, animated: true, completion: nil)
}
}
}
Popular Ios topics
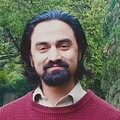
If I install Catalina on unsupported models of MacBook Pro through a patcher, will there be any (certification or other) problem while co...
New
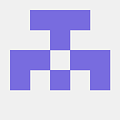
Hi! How effectively caching data of networking in iOS Swift? What libraries, techniques are you use? Can you share good materials(books, ...
New
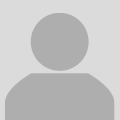
Hi all,
we want to develop an iPhone IOS app that will have 3 sections/uses and we would like your opinion if it can all be done and all...
New
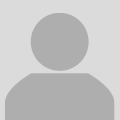
does anyone have experience on swiftui? i was thinking about trying something new, unfortunately since my company is old i am not allowed...
New
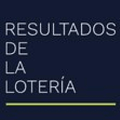
HI,
I am Mehmood khan Computer Science Student from Pakistan and I have a project in client want to made an automate application that wh...
New
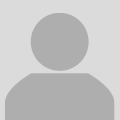
Hi Everyone, I am Emmanuel Katto originally from Uganda. Now living in UK. I am new to this community. I want to know which blogs you peo...
New
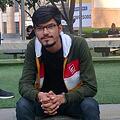
I have a website of a chatbot that also accepts microphone inputs and I embedded that into my swift and the everything other than the mic...
New
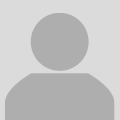
Hey team I’m really wanting a retro i pod feel on my ipad revision 2 running 9.3.5 is there any one out there that can make this work or ...
New
Other popular topics
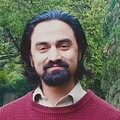
I know that -t flag is used along with -i flag for getting an interactive shell. But I cannot digest what the man page for docker run com...
New
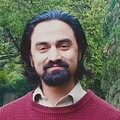
New
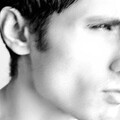
This looks like a stunning keycap set :orange_heart:
A LEGENDARY KEYBOARD LIVES ON
When you bought an Apple Macintosh computer in the e...
New
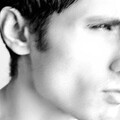
We’ve talked about his book briefly here but it is quickly becoming obsolete - so he’s decided to create a series of 7 podcasts, the firs...
New
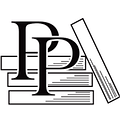
Rails 7 completely redefines what it means to produce fantastic user experiences and provides a way to achieve all the benefits of single...
New
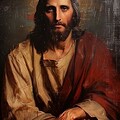
The File System Access API with Origin Private File System.
WebKit supports new API that makes it possible for web apps to create, open,...
New
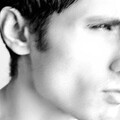
Was just curious to see if any were around, found this one:
I got 51/100:
Not sure if it was meant to buy I am sure at times the b...
New
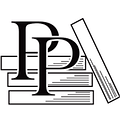
Author Spotlight:
David Bryant Copeland
@davetron5000
We’re so happy to bring you another Author Spotlight, a series where we sit dow...
New
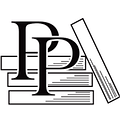
Author Spotlight:
Tammy Coron
@Paradox927
Gaming, and writing games in particular, is about passion, vision, experience, and immersio...
New
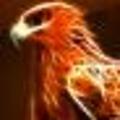
Background
Lately I am in a quest to find a good quality TTS ai generation tool to run locally in order to create audio for some videos I...
New
Categories:
Sub Categories:
Popular Portals
- /elixir
- /rust
- /ruby
- /wasm
- /erlang
- /phoenix
- /keyboards
- /rails
- /js
- /python
- /security
- /go
- /swift
- /vim
- /clojure
- /emacs
- /java
- /haskell
- /onivim
- /svelte
- /typescript
- /crystal
- /c-plus-plus
- /kotlin
- /tailwind
- /gleam
- /react
- /ocaml
- /flutter
- /elm
- /vscode
- /ash
- /opensuse
- /centos
- /php
- /deepseek
- /html
- /scala
- /zig
- /textmate
- /sublime-text
- /debian
- /nixos
- /lisp
- /agda
- /react-native
- /kubuntu
- /arch-linux
- /ubuntu
- /revery
- /manjaro
- /django
- /spring
- /diversity
- /lua
- /nodejs
- /julia
- /slackware
- /c
- /neovim